Although Symfony 2.6.0 was released a few days ago, there are still some new and noteworthy features that haven't been explained in this blog. The most important of those missing features is the addition of a new component called VarDumper.
VarDumper component aims to replace the well-known var_dump()
PHP function
with a more modern and fully-featured alternative called dump()
. Before using
it, make sure that the DebugBundle
bundle is enabled in the application's
kernel:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
// app/AppKernel.php
use Symfony\Component\HttpKernel\Kernel;
use Symfony\Component\Config\Loader\LoaderInterface;
class AppKernel extends Kernel
{
public function registerBundles()
{
$bundles = array(
// ...
);
if (in_array($this->getEnvironment(), array('dev', 'test'))) {
$bundles[] = new Symfony\Bundle\DebugBundle\DebugBundle();
// ...
}
}
// ...
}
Now you can replace all your var_dump()
calls with the new and shorter dump()
function. Unlike var_dump()
, you can safely use dump()
to display the contents
of any variable, including complex variables with circular references such as
Doctrine entities.
Consider for example this controller that dumps the full Symfony container and
the full Request
object:
1 2 3 4 5 6 7 8 9 10 11 12
namespace AppBundle\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\Controller;
class DefaultController extends Controller
{
public function indexAction(Request $request)
{
dump($this->container, $request);
// ...
}
}
When displaying this controller in a browser, you'll see a new panel called
dump
which captures all the dumped variables and shows a preview of their
contents:
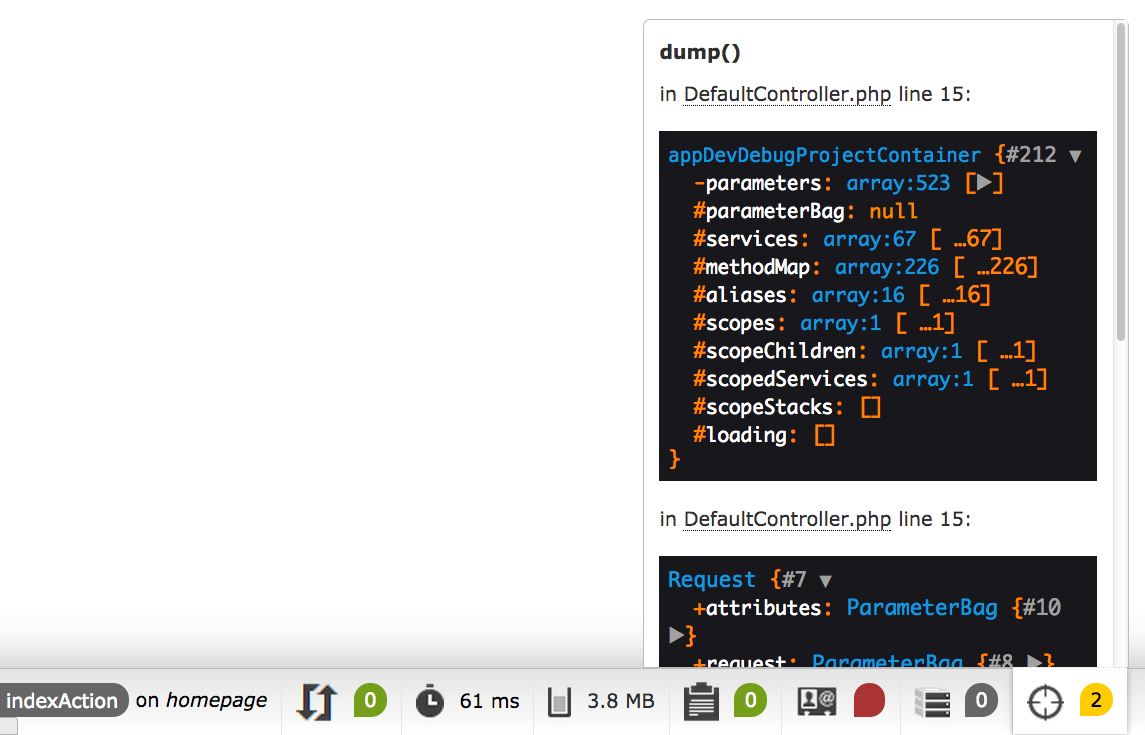
Click on the dumper
panel and you'll get the full detail of those variables,
including information about references, public/protected/private properties and
methods, unlimited nesting level, etc.:
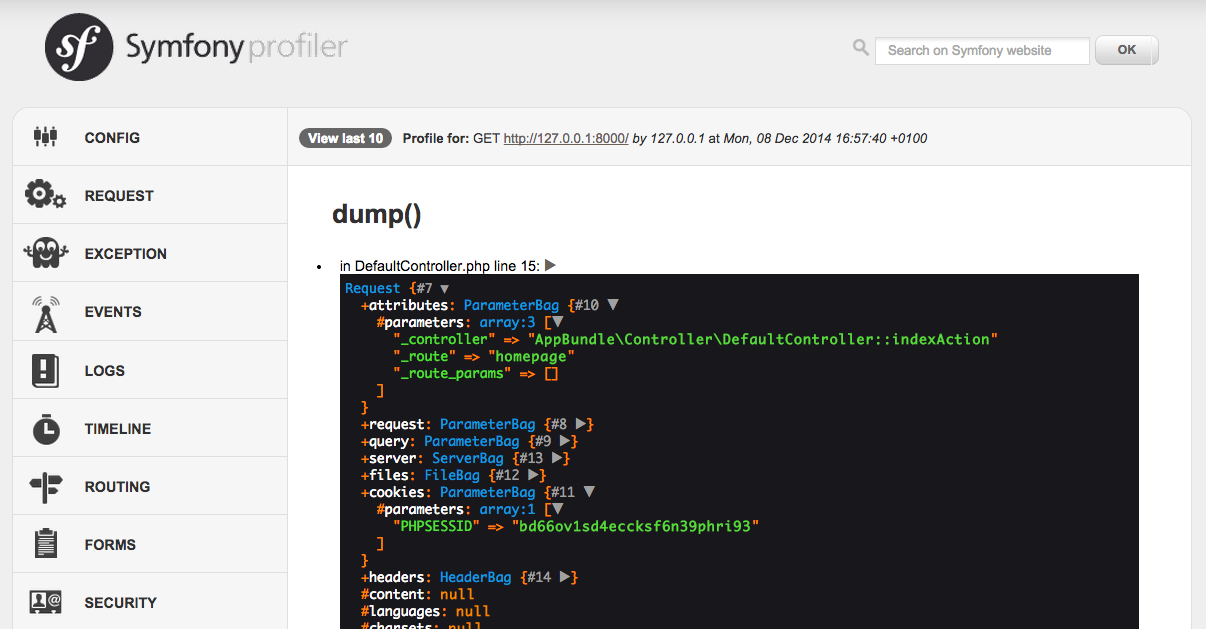
In addition to the seamless integration with the Symfony Web Debug Toolbar, the
component is smart enough to detect if you are using exit
or die()
in your
code. In those cases, variable dumps are written on the regular output.
VarDumper component also includes the {% dump %}
tag and the {{ dump() }}
function for inspecting variables directly from the Twig templates. The {% dump %}
tag dumps variables to the web debug toolbar (e.g {% dump variable1, variable2 %}
),
which is the best alternative when the output of the original template shall not
be modified.
On the contrary, the {{ dump() }}
function dumps variables inline, so their
contents are displayed alongside the regular template contents (e.g
{{ dump(variable1, variable2) }}
).
VarDumper component is natively available since 2.6.0, but if some of your projects
are stuck in Symfony 2.3, 2.4 or 2.5 versions, you can install the backported
debug-bundle
as follows:
1
$ composer require --dev tchwork/debug-bundle
Lastly, don't forget to check out the documentation of VarDumper component, including the advanced Usage of the VarDumper Component article.
Noce! But you should suggest a require --dev in the last part, since likely you're gonna use it in dev only.
*Nice
@Massimiliano you are right! I've just updated the post to add the
--dev
option to the last command. Thanks!This is great !
Grrrreat! :)
According to your blog post, it seems like this Component is tightly tied to the DebugBundle and FrameworkBundle (for the WDT). Can it also be used in standalone in any PHP project easily ?
It's a great feature for people doesn't own xdebug ! Thank you
Very usefull! i used it few days ago! But I didn't know for the Twig part! Thks
Debugger, getting better!
Great Work!
Tested and approved! I love it! Thank you <3
Is there a way to easily dump as string? (in order to write the output in a log file, for instance)
What happen, if I forgot "dump($this->container, $request);" in the production env? Will it fail?
Yes!!! It's gonna be very useful. Thx
--dev or not depends on what you're doing with the component: if you use it only for debugging, fine, but you can also do more (let me know what :) )
The VarDumper component has no external dependency. The DebugBundle & co. is required only for integrating with the WebProfiler toolbar. Here is an integration with Silex for example: https://github.com/jeromemacias/Silex-Debug
To get the dump as a string, you should read: http://symfony.com/doc/current/components/var_dumper/advanced.html#dumpers
Last but not least, if you forgot "dump($this->container, $request);" in the production env, then you'll get a fatal error (we chosed to make it this way to prevent any sensitive data disclosure)
I think, having things, that working only in debug mode is rather strange. I like current "fail-fast" design of most Symfony components, when they fail in dev env and silently fail (or just do nothing) in prod env. I'd rather prefer prod env equivalent of this function, which simply does nothing if kernel.debug is off. If not - I can't even leave this function at the code or have to manually check for debug flag before using it.
soooo, finally get it. this is a must have. thank you very much.
Great feature!
Cool to have this as a standard component.
@Nicolas Grekas : thanks for the tips!
Definitely useful. Many thanks for this piece of code. Makes me happy every day!
Cool!
Cool!
Really cool. (But I would have named it "sfdump" or something like that. Because in the future "dump" could be a new core php function)
Literally cut my Debugging time in half! Great work!