Symfony 2.7 includes countless code tweaks and improvements. A lot of them are minor changes that will increase your day-to-day productivity. In this article we introduce seven of those small but nice features.
Added a getParameter()
shortcut method in the base controller
Contributed by
Hugo Hamon
in #14052.
Getting the value of a container parameter inside a controller is one of the
most common frustrations when you start learning Symfony. You usually try to use
the get()
method first with no luck and then you try to use the inexistent
getParameter()
method. Luckily, Symfony 2.7 will add a new getParameter()
shortcut:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
use Symfony\Bundle\FrameworkBundle\Controller\Controller;
class DefaultController extends Controller
{
public function indexAction()
{
// Symfony 2.6
$value = $this->container->getParameter('param_name');
// Symfony 2.7
$value = $this->getParameter('param_name');
// ...
}
}
Added feedback about the Symfony version status
Contributed by
Wouter De Jong
in #13626.
Who wants to run an outdated Symfony version considering the great features introduced in each new release? Fortunately, starting from Symfony 2.7, it will be harder to run an outdated version. The reason is that the web debug toolbar will include a visual feedback about the status of your Symfony version:
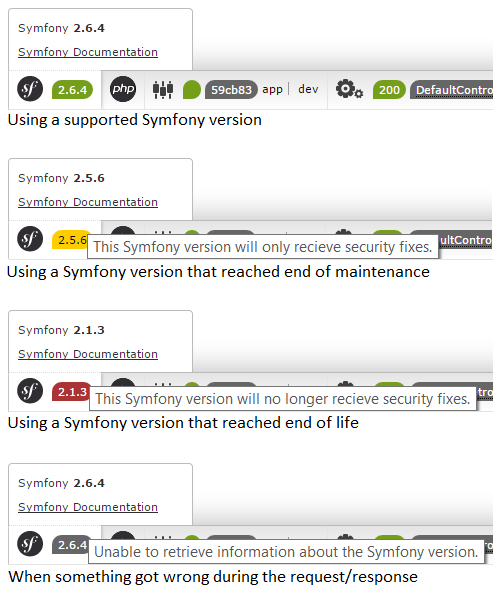
Displayed the HTTP status in profiler search results
Contributed by
Alexander Schwenn
in #13034.
Sometimes it's hard to look for a specific request among all the requests stored by the Symfony profiler. In Symfony 2.7 this will be a bit easier thanks to the new column that displays the HTTP status of each request.
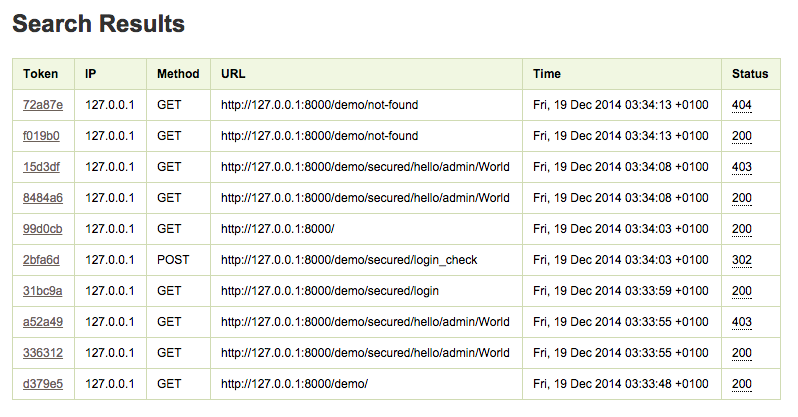
Please note that if you store the profiler information in a database of type MySQL
or SQLite, you must drop the existing table or add the new status_code
column
manually.
Add support for container parameters in route conditions
Contributed by
Nikita Nefedov
in #12960.
In Symfony 2.7, route conditions now support using container parameters in their
expressions. Just wrap the parameter names with %
characters and Symfony will
replace them by their values before evaluating the expression:
1 2 3 4 5
# app/config/routing.yml
contact:
path: /contact
defaults: { _controller: AcmeDemoBundle:Main:contact }
condition: "request.headers.get('User-Agent') matches '%allowed_user_agents%'"
Please note that this change introduces a minor BC break in some edge cases. If
your expression uses the modulo operator in this way: foo%bar%2
, Symfony 2.6
will parse it as $foo % $bar % 2
and Symfony 2.7 will replace %bar%
by
the value of the bar
container parameter or it will raise an error if it
doesn't exist.
Moved lint commands to the lint:
namespace
Contributed by
Abdellatif Ait boudad
in #14116.
In the past we moved all Symfony debug commands to the debug:
namespace in
order to better group commands by function. Symfony 2.7 does the same with the
lint commands used to discover syntax errors in YAML files and Twig templates:
1 2 3 4 5 6 7
# Symfony 2.6
$ php app/console yaml:lint ...
$ php app/console twig:lint ...
# Symfony 2.7 (the old command names also work)
$ php app/console lint:yaml ...
$ php app/console lint:twig ...
This may seem as a negligible change, but grouping debug commands under debug:
and lint commands under lint:
makes the framework more consistent and that's
always a good thing.
Add support for linting multiple Twig templates
Contributed by
Miroslav Šustek
in #13548.
In Symfony 2.7 you can pass any number of files and/or directories to the
lint:twig
command:
1 2 3 4 5 6 7 8 9 10
# Symfony 2.6 (one template or one directory)
$ php app/console lint:twig app/Resources/views/base.html.twig
1/1 valid files
$ php app/console lint:twig app/Resources/views/blog/
4/4 valid files
# Symfony 2.7 (any number of files and/or directories)
$ php app/console lint:twig app/Resources/views/base.html.twig app/Resources/views/blog/
5/5 valid files
Automatically restart the built-in web server
Contributed by
Wouter De Jong
in #14198.
Using the PHP built-in web server is increasingly common while developing applications.
You probably use the server:run
command available since Symfony 2.2. However,
starting from Symfony 2.6 you can also use the server:start
, server:status
and server:stop
commands.
The only drawback is that the server:start
command requires the pcntl
extension to run. In Symfony 2.7, if you execute server:start
and you don't
have the pcntl
extension, Symfony will automatically execute the
server:run
command.
Be an active member of the community
All these improvements have been proposed and implemented by the amazing Symfony Community. If you also want to become an active community member, read the Symfony contributing guides and consider proposing new ideas, sending pull requests and reviewing the code submitted by other developers.
Glad to see the getParameter method appareance, but wouldn't it be a good idea to allow a default value for a parameter if it hasn't been defined just like the get method of the Request object ?
Doing so, you could ensure a consistent behaviour wether the parameter is set or not.
HTH
@Joachim, in the discussion related to the new getParameter() method you can see the details about why we didn't add that feature: https://github.com/symfony/symfony/pull/14052
In short, it makes sense to provide a default value for a request query parameter because they are optional, but it doesn't make sense for the container parameters because most of the times you want them to define a value or make the application fail fast to alert you.
These are small but amazing changes :) looking forward to use them!
@Javier, thanks for the link and your explanation, I now understand why it's not implemented.
I suggested this because of a project I'm currently working on which has options that can be defined depending on project instances. By the way, in this project, I created my own method getParameterIfDefined which just do what I need.
Seems to me there's a typo in the screenshots : "recieve" instead of "receive".
You nailed it team!!!
Where can I see all the requests for new Symfony features and related messages from members with suggestions?
@Gonçalo You can browse and search open and closed issues on GitHub: https://github.com/symfony/symfony/issues
Thanx! Lazy as we are, i already love the getParameter shortcut :D
Thanks