Cursor Helper
Warning: You are browsing the documentation for Symfony 6.0, which is no longer maintained.
Read the updated version of this page for Symfony 7.2 (the current stable version).
Cursor Helper
The Cursor allows you to change the cursor position in a console command. This allows you to write on any position of the output:
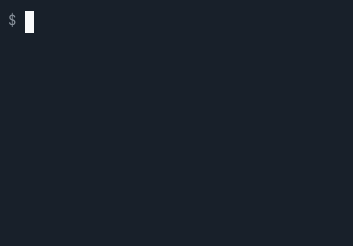
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
// src/Command/MyCommand.php
namespace App\Command;
use Symfony\Component\Console\Command\Command;
use Symfony\Component\Console\Cursor;
use Symfony\Component\Console\Input\InputInterface;
use Symfony\Component\Console\Output\OutputInterface;
class MyCommand extends Command
{
// ...
public function execute(InputInterface $input, OutputInterface $output): int
{
// ...
$cursor = new Cursor($output);
// moves the cursor to a specific column (1st argument) and
// row (2nd argument) position
$cursor->moveToPosition(7, 11);
// and write text on this position using the output
$output->write('My text');
// ...
}
}
Using the cursor
Moving the cursor
There are few methods to control moving the command cursor:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
// moves the cursor 1 line up from its current position
$cursor->moveUp();
// moves the cursor 3 lines up from its current position
$cursor->moveUp(3);
// same for down
$cursor->moveDown();
// moves the cursor 1 column right from its current position
$cursor->moveRight();
// moves the cursor 3 columns right from its current position
$cursor->moveRight(3);
// same for left
$cursor->moveLeft();
// move the cursor to a specific (column, row) position from the
// top-left position of the terminal
$cursor->moveToPosition(7, 11);
You can get the current command's cursor position by using:
1 2 3
$position = $cursor->getCurrentPosition();
// $position[0] // columns (aka x coordinate)
// $position[1] // rows (aka y coordinate)
Clearing output
The cursor can also clear some output on the screen:
1 2 3 4 5 6 7 8 9 10 11
// clears all the output from the current line
$cursor->clearLine();
// clears all the output from the current line after the current position
$cursor->clearLineAfter();
// clears all the output from the cursors' current position to the end of the screen
$cursor->clearOutput();
// clears the entire screen
$cursor->clearScreen();
You also can leverage the show() and hide() methods on the cursor.
This work, including the code samples, is licensed under a
Creative Commons BY-SA 3.0 license.