The New in Symfony 7.2 blog series showcases the main new features introduced in Symfony 7.2. This two-part blog post is the last in the series and highlights some miscellaneous minor changes and improvements.
Custom Message Retry Delay
When using the Messenger component, you can throw a RecoverableMessageHandlingException
to force retrying a message an unlimited number of times. In Symfony 7.2,
you can pass a custom retry delay to the constructor of that exception. This is
useful, for example, when retrying an HTTP request that included a Retry-After
response header.
Better Coalesce Support in Expressions
The ExpressionLanguage component supports the null-coalesce operator (foo ?? 'no'
,
foo.baz ?? foo['baz'] ?? 'no'
, etc.) However, unlike PHP's equivalent operator,
it throws an exception when trying to access a non-existent variable.
We improved this in Symfony 7.2, and the null-coalesce operator now behaves exactly the same as the PHP operator.
Passing Custom Attributes to Passports via User Login
When using the login()
method to login users programmatically, you can pass
different options:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
use Symfony\Bundle\SecurityBundle\Security;
// ...
class SomeController
{
public function someAction(Security $security): Response
{
// ...
// log the user in on the current firewall
$security->login($user);
// log in on a different firewall...
$security->login($user, 'form_login', 'other_firewall');
// add badges...
$security->login($user, 'form_login', 'other_firewall', [(new RememberMeBadge())->enable()]);
// ...
}
}
In Symfony 7.2, you can also define custom attributes to pass to the passport:
1 2
// the attributes will be added to the user passport
$security->login($user, attributes: ['referer' => 'https://oauth.example.com']);
Add calendar
Option to DateType
In PHP, the IntlCalendar class allows you to select any of the calendars defined
in the ICU library and also define your own calendar tweaks. In Symfony 7.2,
we've improved the DateType form field to allow passing a custom calendar via
the new calendar
option.
Force Color in Console Output
In Symfony 4.4, we added support for the NO_COLOR env var as a way to disable
console output coloring in an industry-standard manner. In Symfony 7.2, we're adding
support for the opposite env var called FORCE_COLOR
.
Set this env var with a non-empty value, and components like Console, PhpUnitBridge, and VarDumper will output colored content.
Support Virtual Properties in VarDumper
One of the main new features of PHP 8.4 is property hooks, which allow class properties to define additional logic associated with their get and set operations:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
class VirtualHookedClass
{
public string $firstName = 'John';
private string $lastName = 'Doe';
public string $fullName {
get {
return $this->firstName.' '.$this->lastName;
}
}
private $untypedFullName {
get {
return $this->firstName.' '.$this->lastName;
}
}
}
In Symfony 7.2, we added support for property hooks when using the VarDumper component to dump the object contents. While the hook value won't be shown (to avoid running the logic inside it), you'll see their names and types:
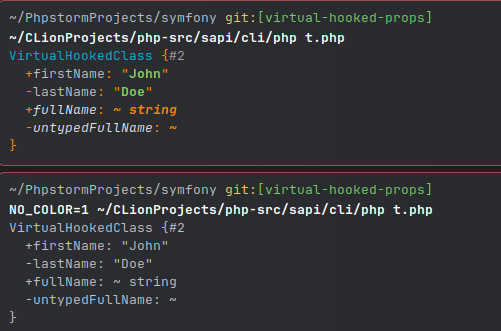