The VarDumper Component
Warning: You are browsing the documentation for Symfony 2.x, which is no longer maintained.
Read the updated version of this page for Symfony 7.2 (the current stable version).
The VarDumper component provides mechanisms for walking through any arbitrary PHP variable. Built on top, it provides a better
dump()
function that you can use instead of var_dump.
Installation
1
$ composer require symfony/var-dumper --dev
Alternatively, you can clone the https://github.com/symfony/var-dumper repository.
Note
If you install this component outside of a Symfony application, you must
require the vendor/autoload.php
file in your code to enable the class
autoloading mechanism provided by Composer. Read
this article for more details.
Note
If using it inside a Symfony application, make sure that the
DebugBundle is enabled in your app/AppKernel.php
file.
The dump() Function
The VarDumper component creates a global dump()
function that you can
use instead of e.g. var_dump. By using it, you'll gain:
- Per object and resource types specialized view to e.g. filter out Doctrine internals while dumping a single proxy entity, or get more insight on opened files with stream_get_meta_data;
- Configurable output formats: HTML or colored command line output;
- Ability to dump internal references, either soft ones (objects or
resources) or hard ones (
=&
on arrays or objects properties). Repeated occurrences of the same object/array/resource won't appear again and again anymore. Moreover, you'll be able to inspect the reference structure of your data; - Ability to operate in the context of an output buffering handler.
For example:
1 2 3 4 5 6
require __DIR__.'/vendor/autoload.php';
// create a variable, which could be anything!
$someVar = ...;
dump($someVar);
By default, the output format and destination are selected based on your current PHP SAPI:
- On the command line (CLI SAPI), the output is written on
STDOUT
. This can be surprising to some because this bypasses PHP's output buffering mechanism; - On other SAPIs, dumps are written as HTML in the regular output.
Note
If you want to catch the dump output as a string, please read the advanced documentation which contains examples of it. You'll also learn how to change the format or redirect the output to wherever you want.
Tip
In order to have the dump()
function always available when running
any PHP code, you can install it globally on your computer:
- Run
composer global require symfony/var-dumper
; - Add
auto_prepend_file = ${HOME}/.composer/vendor/autoload.php
to yourphp.ini
file; - From time to time, run
composer global update symfony/var-dumper
to have the latest bug fixes.
DebugBundle and Twig Integration
The DebugBundle allows greater integration of the component into the Symfony full-stack framework. It is enabled by default in the dev and test environment of the Symfony Standard Edition.
Since generating (even debug) output in the controller or in the model
of your application may just break it by e.g. sending HTTP headers or
corrupting your view, the bundle configures the dump()
function so that
variables are dumped in the web debug toolbar.
But if the toolbar cannot be displayed because you e.g. called die
/exit
or a fatal error occurred, then dumps are written on the regular output.
In a Twig template, two constructs are available for dumping a variable. Choosing between both is mostly a matter of personal taste, still:
{% dump foo.bar %}
is the way to go when the original template output shall not be modified: variables are not dumped inline, but in the web debug toolbar;- on the contrary,
{{ dump(foo.bar) }}
dumps inline and thus may or not be suited to your use case (e.g. you shouldn't use it in an HTML attribute or a<script>
tag).
This behavior can be changed by configuring the debug.dump_destination
option. Read more about this and other options in
the DebugBundle configuration reference.
Using the VarDumper Component in your PHPUnit Test Suite
2.7
The VarDumperTestTrait was introduced in Symfony 2.7.
The VarDumper component provides a trait that can help writing some of your tests for PHPUnit.
This will provide you with two new assertions:
- assertDumpEquals()
- verifies that the dump of the variable given as the second argument matches the expected dump provided as a string in the first argument.
- assertDumpMatchesFormat()
-
is like the previous method but accepts placeholders in the expected dump,
based on the
assertStringMatchesFormat()
method provided by PHPUnit.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
use PHPUnit\Framework\TestCase;
class ExampleTest extends TestCase
{
use \Symfony\Component\VarDumper\Test\VarDumperTestTrait;
public function testWithDumpEquals()
{
$testedVar = array(123, 'foo');
$expectedDump = <<<EOTXT
array:2 [
0 => 123
1 => "foo"
]
EOTXT;
$this->assertDumpEquals($expectedDump, $testedVar);
}
}
Tip
If you still use PHP 5.3, you can extend the VarDumperTestClass instead.
Dump Examples and Output
For simple variables, reading the output should be straightforward. Here are some examples showing first a variable defined in PHP, then its dump representation:
1 2 3 4 5 6 7 8
$var = array(
'a simple string' => "in an array of 5 elements",
'a float' => 1.0,
'an integer' => 1,
'a boolean' => true,
'an empty array' => array(),
);
dump($var);

Note
The gray arrow is a toggle button for hiding/showing children of nested structures.
1 2 3 4 5 6 7
$var = "This is a multi-line string.\n";
$var .= "Hovering a string shows its length.\n";
$var .= "The length of UTF-8 strings is counted in terms of UTF-8 characters.\n";
$var .= "Non-UTF-8 strings length are counted in octet size.\n";
$var .= "Because of this `\xE9` octet (\\xE9),\n";
$var .= "this string is not UTF-8 valid, thus the `b` prefix.\n";
dump($var);

1 2 3 4 5 6 7 8 9
class PropertyExample
{
public $publicProperty = 'The `+` prefix denotes public properties,';
protected $protectedProperty = '`#` protected ones and `-` private ones.';
private $privateProperty = 'Hovering a property shows a reminder.';
}
$var = new PropertyExample();
dump($var);

Note
`#14` is the internal object handle. It allows comparing two consecutive dumps of the same object.
1 2 3 4 5 6 7 8
class DynamicPropertyExample
{
public $declaredProperty = 'This property is declared in the class definition';
}
$var = new DynamicPropertyExample();
$var->undeclaredProperty = 'Runtime added dynamic properties have `"` around their name.';
dump($var);

1 2 3 4 5 6 7
class ReferenceExample
{
public $info = "Circular and sibling references are displayed as `#number`.\nHovering them highlights all instances in the same dump.\n";
}
$var = new ReferenceExample();
$var->aCircularReference = $var;
dump($var);

1 2 3 4 5 6 7 8
$var = new \ErrorException(
"For some objects, properties have special values\n"
."that are best represented as constants, like\n"
."`severity` below. Hovering displays the value (`2`).\n",
0,
E_WARNING
);
dump($var);
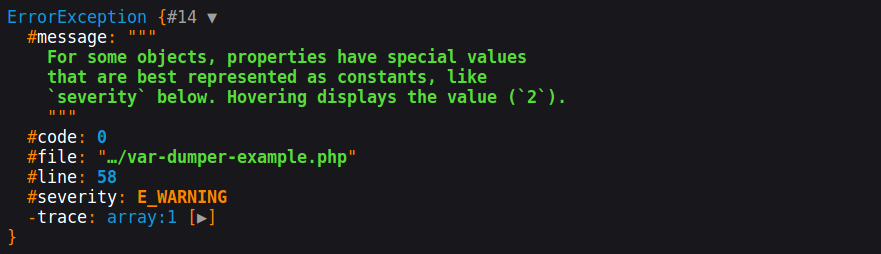
1 2 3 4 5 6 7 8
$var = array();
$var[0] = 1;
$var[1] =& $var[0];
$var[1] += 1;
$var[2] = array("Hard references (circular or sibling)");
$var[3] =& $var[2];
$var[3][] = "are dumped using `&number` prefixes.";
dump($var);
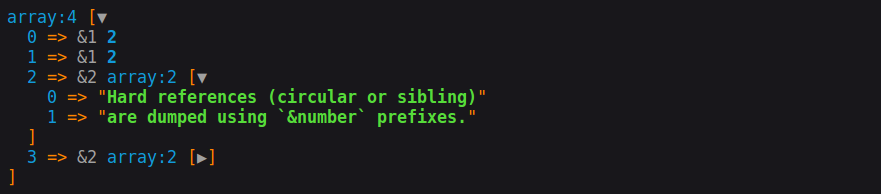
1 2 3 4 5
$var = new \ArrayObject();
$var[] = "Some resources and special objects like the current";
$var[] = "one are sometimes best represented using virtual";
$var[] = "properties that describe their internal state.";
dump($var);
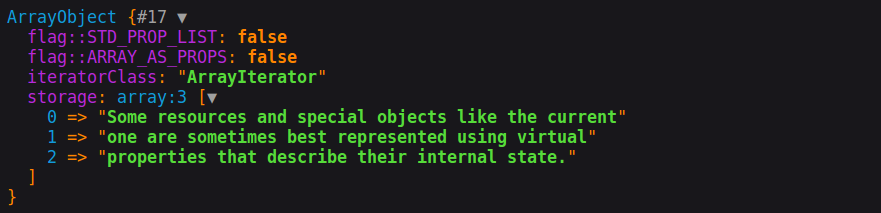
1 2 3 4 5 6 7 8
$var = new AcmeController(
"When a dump goes over its maximum items limit,\n"
."or when some special objects are encountered,\n"
."children can be replaced by an ellipsis and\n"
."optionally followed by a number that says how\n"
."many have been removed; `9` in this case.\n"
);
dump($var);
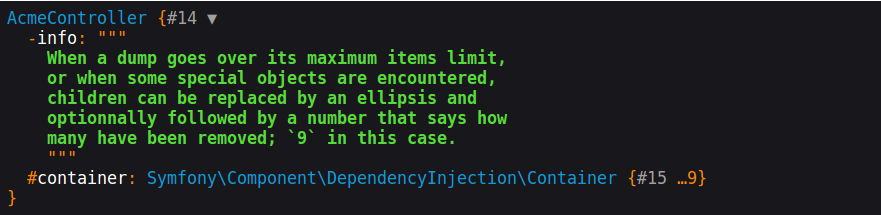