How to Dump Workflows
Warning: You are browsing the documentation for Symfony 6.1, which is no longer maintained.
Read the updated version of this page for Symfony 7.3 (the current stable version).
How to Dump Workflows
To help you debug your workflows, you can generate a visual representation of them as SVG or PNG images. First, install any of these free and open source applications needed to generate the images:
- Graphviz, provides the
dot
command; - Mermaid CLI, provides the
mmdc
command; - PlantUML, provides the
plantuml.jar
file (which requires Java).
If you are defining the workflow inside a Symfony application, run this command to dump it as an image:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
# using Graphviz's 'dot' and SVG images
$ php bin/console workflow:dump workflow-name | dot -Tsvg -o graph.svg
# using Graphviz's 'dot' and PNG images
$ php bin/console workflow:dump workflow-name | dot -Tpng -o graph.png
# using PlantUML's 'plantuml.jar'
$ php bin/console workflow:dump workflow_name --dump-format=puml | java -jar plantuml.jar -p > graph.png
# highlight 'place1' and 'place2' in the dumped workflow
$ php bin/console workflow:dump workflow-name place1 place2 | dot -Tsvg -o graph.svg
# using Mermaid.js CLI
$ php bin/console workflow:dump workflow_name --dump-format=mermaid | mmdc -o graph.svg
The DOT image will look like this:
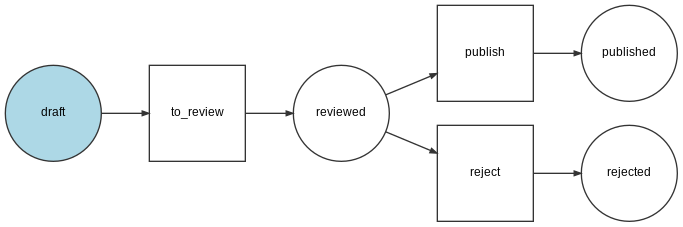
The Mermaid image will look like this:
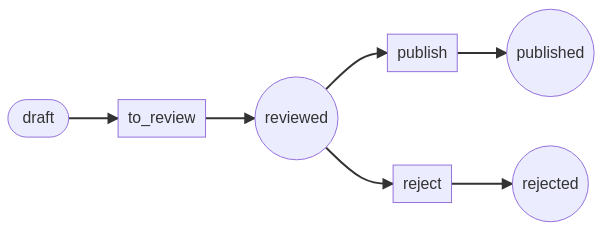
The PlantUML image will look like this:
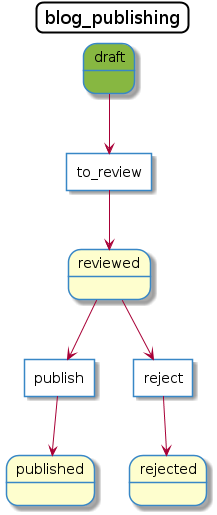
If you are creating workflows outside of a Symfony application, use the
GraphvizDumper
or StateMachineGraphvizDumper
class to create the DOT
files and PlantUmlDumper
to create the PlantUML files:
1 2 3 4 5 6 7
// Add this code to a PHP script; for example: dump-graph.php
$dumper = new GraphvizDumper();
echo $dumper->dump($definition);
# if you prefer PlantUML, use this code:
# $dumper = new PlantUmlDumper();
# echo $dumper->dump($definition);
1 2 3
# replace 'dump-graph.php' by the name of your PHP script
$ php dump-graph.php | dot -Tsvg -o graph.svg
$ php dump-graph.php | java -jar plantuml.jar -p > graph.png
Styling
You can use metadata
with the following keys to style the workflow:
for places:
bg_color
: a color;description
: a string that describes the state.
for transitions:
label
: a string that replaces the name of the transition;color
: a color;arrow_color
: a color.
Strings can include \n
characters to display the contents in multiple lines.
Colors can be defined as:
- a color name from PlantUML's color list;
- an hexadecimal color (both
#AABBCC
and#ABC
formats are supported).
Note
The Mermaid dumper does not support coloring the arrow heads
with arrow_color
as there is no support in Mermaid for doing so.
Below is the configuration for the pull request state machine with styling added.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
# config/packages/workflow.yaml
framework:
workflows:
pull_request:
type: 'state_machine'
marking_store:
type: 'method'
property: 'currentPlace'
supports:
- App\Entity\PullRequest
initial_marking: start
places:
start: ~
coding: ~
test: ~
review:
metadata:
description: Human review
merged: ~
closed:
metadata:
bg_color: DeepSkyBlue
transitions:
submit:
from: start
to: test
update:
from: [coding, test, review]
to: test
metadata:
arrow_color: Turquoise
wait_for_review:
from: test
to: review
metadata:
color: Orange
request_change:
from: review
to: coding
accept:
from: review
to: merged
metadata:
label: Accept PR
reject:
from: review
to: closed
reopen:
from: closed
to: review
The PlantUML image will look like this:
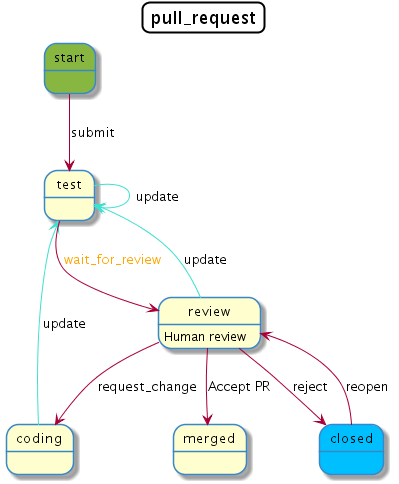