The VarDumper component provides a dump()
function as a more advanced
alternative to PHP's var_dump()
function. The problem of dumping data from
your application is that, for example, when working on an API you might end up
in the console with a mix of your response data and the dumped data:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
class ApiController extends AbstractController
{
/**
* @Route("/hello")
*/
public function hello(Request $request, UserInterface $user)
{
dump($request->attributes, $user);
return JsonResponse::create([
'status' => 'OK',
'message' => "Hello {$user->getUsername()}"
]);
}
}
In this case, the console output is confusing:
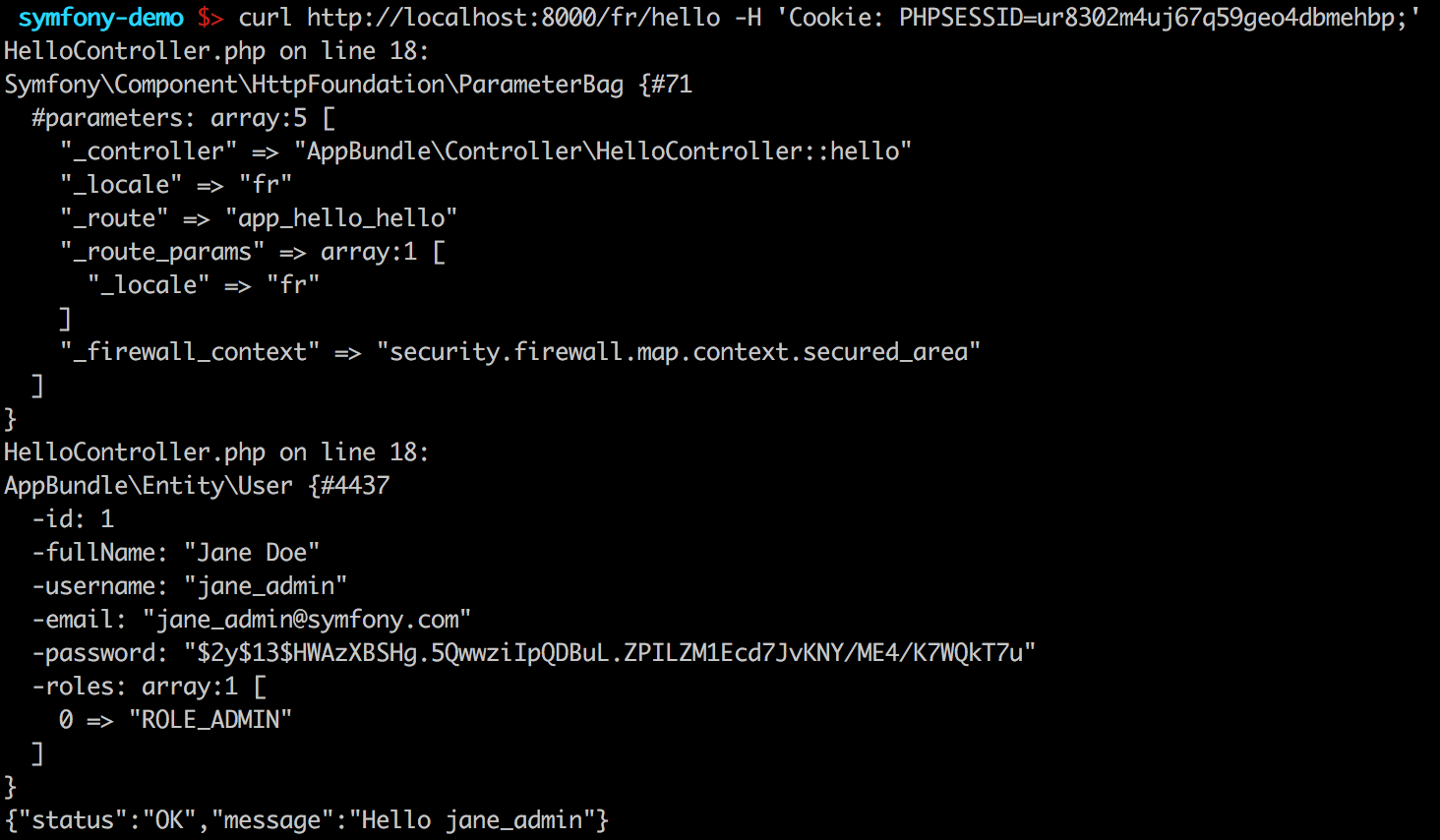
In order to solve these issues, in Symfony 4.1 we've introduced a dedicated
server to collect the dumped data. In practice you just need to run the new
server:dump
command and whenever you call to dump()
, the dumped data
is sent to a single centralized server that outputs it to the console or to a
file in HTML format:
1 2 3 4 5 6
# displays the dumped data in the console:
$ ./bin/console server:dump
[OK] Server listening on tcp://0.0.0.0:9912
# stores the dumped data in a file using the HTML format:
$ ./bin/console server:dump --format=html > dump.html
This is how the server looks when dumping contents to the console (which includes context information such as the source file, the HTTP request, the executed command, etc.):
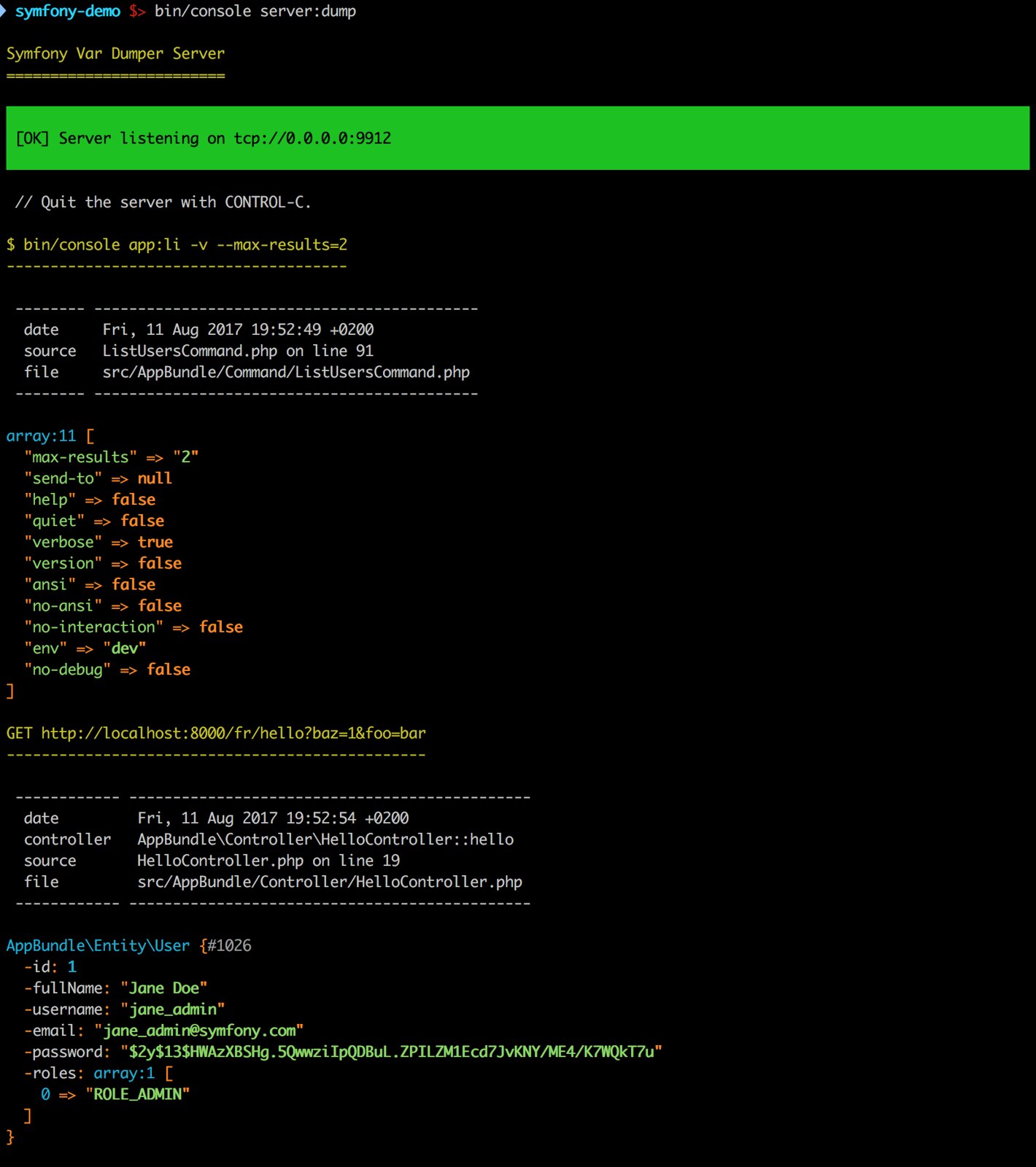
And this is how it looks when using the HTML format:
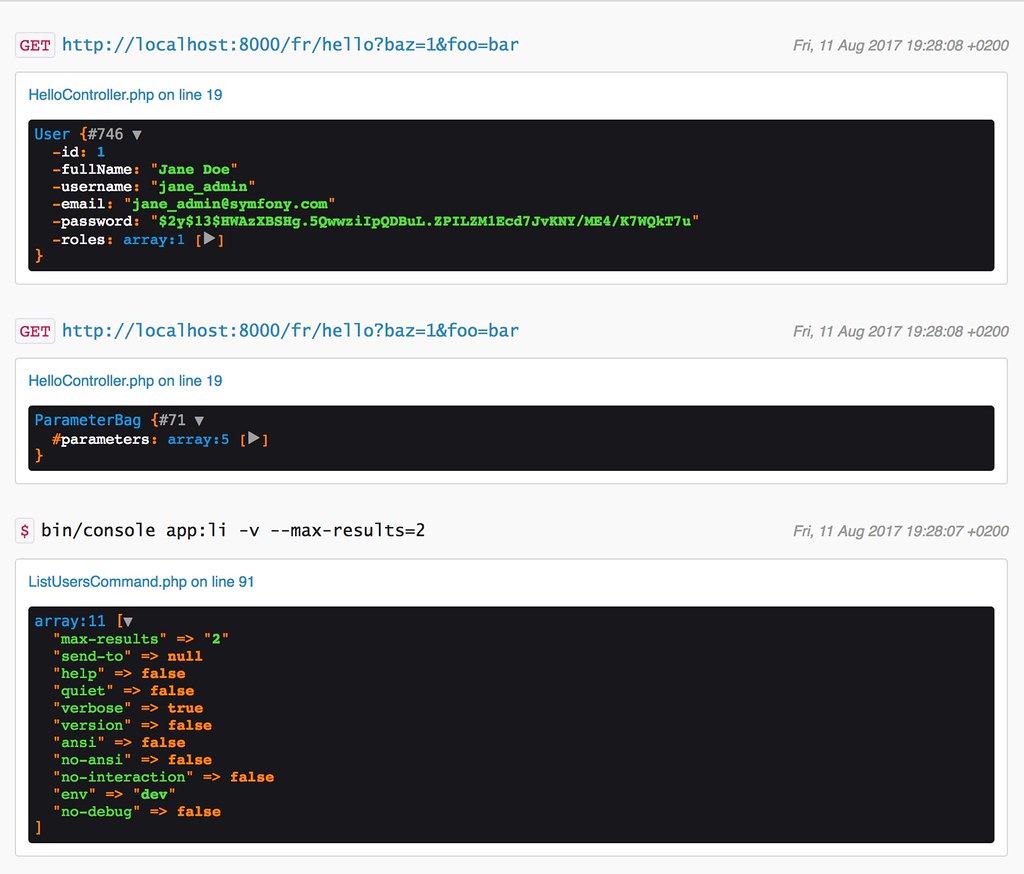
When using it inside a Symfony application, the new server is configured in the
debug
package:
1 2 3
# config/packages/dev/debug.yaml
debug:
dump_destination: "tcp://%env(VAR_DUMPER_SERVER)%"
Great!!!!
Kudos!!!
Awesome! Will help a lot!! Thanks!
Wow great ! Will it be possible to launch many instance of different ports ?
@Guillaume Le Bigot: Sure. Just use another value for the
debug.dump_destination
. Or start the server by prepending the command with a different ip/port for theVAR_DUMPER_SERVER
var (default is set by the DebugBundle to 127.0.0.1:9912), i.e:$> VAR_DUMPER_SERVER="127.0.0.1:9913" bin/console server:dump
and for the server dumper to send Data clones to the right server, start the php web server with the same env var value:
$> VAR_DUMPER_SERVER="127.0.0.1:9913" bin/console server:run
But actually, the idea of the env var is more to help centralizing dumps to a single server instance when working with multiple apps, so you have only this env var to tweak globally.
Awesome!
That the kind of stuff I love. Great work @Maxime! <3
Interesting looks!
Looks better then some actual website design I've seen :} Really nice!
Nice job, looks useful!
Just... awesome!
Thanks!
Awesome !
There is also an super project here https://github.com/omouren/external-var-dumper-bundle to use a ViewJS UI to watch dumps.
This is super useful and makes the DX so much better! What a great idea!
This will save developers lot of searching time ;)
Might not be worth an update, but for future readers: the component itself is now shipped with php binary script, so you can start a server easily at any time by executing
vendor/bin/var-dump-server
as soon as you have the "symfony/var-dumper" and "symfony/console" components.A PR is also pending to make registration of the ServerDumper easy: https://github.com/symfony/symfony/pull/26695