Access to the Estimated Password Strength
The PasswordStrength constraint validates that the given password has reached
a minimum strength configured in the constraint. In Symfony 7.2, we've changed
the visibility of the estimateStrength()
validator method from private to public.
This allows you to access the estimated password strength and display it, for example, in the interface, so users can better understand the quality of their passwords.
Simpler RequestStack Unit Testing
When using the RequestStack in unit tests, you previously needed code like this to configure the requests:
1 2 3
$requestStack = new RequestStack();
$requestStack->push(Request::create('/'));
$someCustomClass = new MyCustomClass($requestStack);
In Symfony 7.2, we've simplified this by adding a constructor to RequestStack
that accepts an array of requests:
1 2 3
$someCustomClass = new MyCustomClass(new RequestStack([
Request::create('/'),
]));
Default Action in the HTML Sanitizer
In Symfony 7.2, we've added a new defaultAction()
method in the HtmlSanitizer component.
This method sets the default action for elements that are not explicitly allowed
or blocked:
1 2 3 4 5 6 7 8
use Symfony\Component\HtmlSanitizer\HtmlSanitizer;
use Symfony\Component\HtmlSanitizer\HtmlSanitizerAction;
$config = (new HtmlSanitizerConfig())
->defaultAction(HtmlSanitizerAction::Block)
->allowElement('p');
$sanitizer = new HtmlSanitizer($config);
HtmlSanitizerAction
is a PHP enum with three cases: Drop
(removes the element
and its children); Block
(removes the element but keeps its children); and Allow
(keeps the element).
Allow Using defaultNull()
on Boolean Nodes
The current defaultNull()
of BooleanNode
, used when
defining and processing configuration values, casts null values to true
.
In Symfony 7.2, we've updated this method so you can define nullable boolean
values properly:
1
->booleanNode('enabled')->defaultNull()->end()
Better IP Address Anonymization
The IpUtils
class includes an anonymize()
method to obscure part of the IP
address for user privacy. In Symfony 7.2, we've added two new arguments to this
method so you can specify how many bytes to anonymize:
1 2 3 4 5 6 7 8 9 10 11 12 13
use Symfony\Component\HttpFoundation\IpUtils;
$ipv4 = '123.234.235.236';
// for IPv4 addresses, you can hide 0 to 4 bytes
$anonymousIpv4 = IpUtils::anonymize($ipv4, 3);
// $anonymousIpv4 = '123.0.0.0'
$ipv6 = '2a01:198:603:10:396e:4789:8e99:890f';
// for IPv6 addresses, you can hide 0 to 16 bytes
// (you must define the second argument (bytes to anonymize in IPv4 addresses)
// even when you are only anonymizing IPv6 addresses)
$anonymousIpv6 = IpUtils::anonymize($ipv6, 3, 10);
// $anonymousIpv6 = '2a01:198:603::'
String Configuration Node
When defining a configuration tree, you can use many node types for
configuration values (boolean, integers, floats, enums, arrays, etc.). However,
you couldn't define string values directly; they were specified as scalar
nodes.
In Symfony 7.2, we've added a string node type and a stringNode()
method,
allowing you to define configuration values as strings explicitly:
1 2 3 4 5 6 7 8 9 10 11
$rootNode
->children()
// ...
->stringNode('username')
->defaultValue('root')
->end()
->stringNode('password')
->defaultValue('root')
->end()
->end()
;
Security Profiler Improvements
In Symfony 7.2, the security panel of the Symfony Profiler has been improved with several new features. First, the authenticators tab has been updated. Previously, authenticators that didn't support the request were not shown:

Now, to make debugging easier, you can see all the application's authenticators. If an authenticator doesn't support the request, it will be labeled as "not supported":

When using a stateful firewall, the token tab of de-authenticated users now includes a link to the request that contained the previously authenticated user:

The authenticators tab has also been redesigned to display information more
clearly. It now also shows whether an authenticator is lazy and includes any exception
passed to the onAuthenticationFailure()
method:
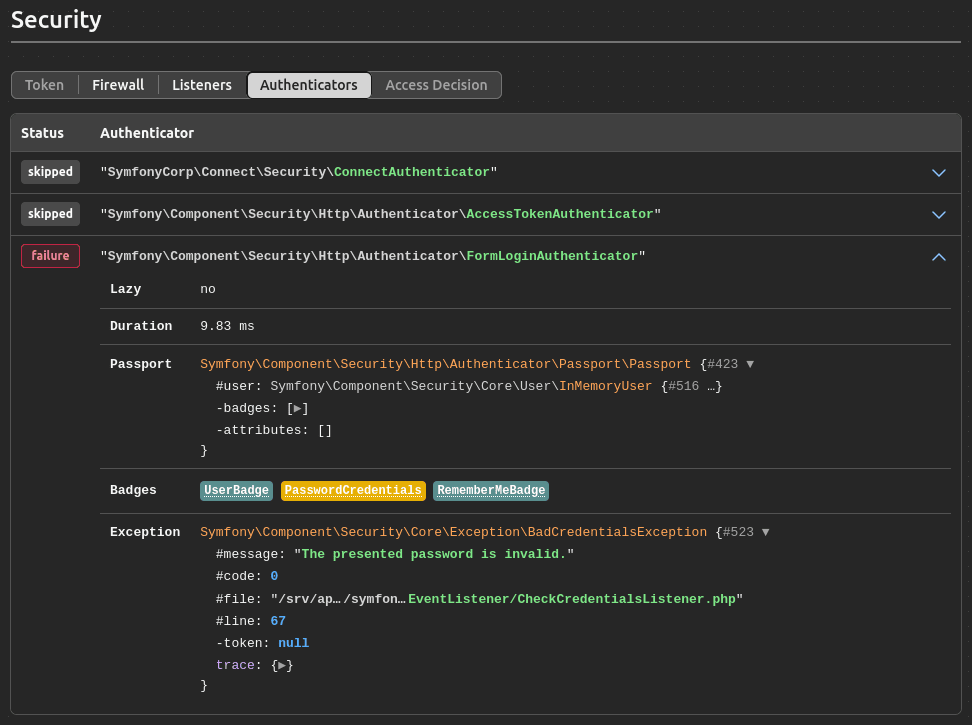
This is the final blog post in the New in Symfony 7.2 series. We hope you enjoyed it and discovered some of the great new features introduced in Symfony 7.2. Check out the Symfony minor version upgrade guide to learn how to upgrade to 7.2 from other 7.x versions. Meanwhile, we've already started working on Symfony 7.3, which will be released at the end of May 2025.